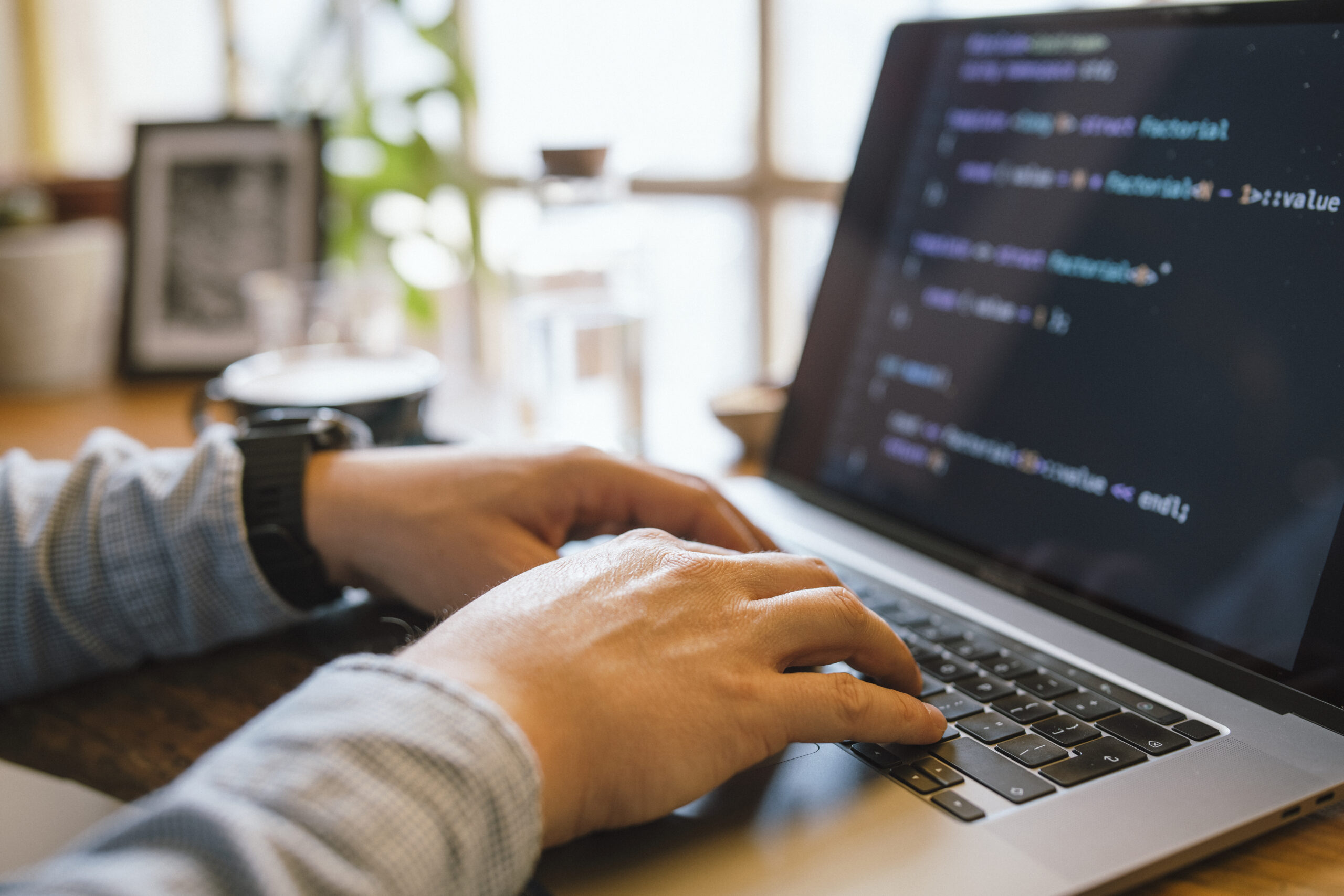
Debugging is One of the more crucial — nonetheless often ignored — capabilities in a very developer’s toolkit. It isn't really pretty much correcting damaged code; it’s about comprehending how and why items go Mistaken, and Mastering to Assume methodically to unravel challenges successfully. Whether you are a starter or simply a seasoned developer, sharpening your debugging competencies can help you save hours of aggravation and significantly enhance your productivity. Here are quite a few procedures that can help builders degree up their debugging recreation by me, Gustavo Woltmann.
Master Your Tools
One of the fastest approaches developers can elevate their debugging skills is by mastering the applications they use on a daily basis. Even though composing code is 1 part of enhancement, figuring out the way to interact with it successfully for the duration of execution is Similarly critical. Modern day improvement environments come Geared up with strong debugging capabilities — but numerous builders only scratch the floor of what these instruments can perform.
Acquire, one example is, an Integrated Advancement Atmosphere (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications enable you to set breakpoints, inspect the worth of variables at runtime, stage through code line by line, and perhaps modify code to the fly. When applied appropriately, they let you notice particularly how your code behaves in the course of execution, which happens to be priceless for monitoring down elusive bugs.
Browser developer resources, for instance Chrome DevTools, are indispensable for front-conclude builders. They let you inspect the DOM, observe network requests, watch real-time effectiveness metrics, and debug JavaScript in the browser. Mastering the console, sources, and community tabs can flip discouraging UI concerns into workable tasks.
For backend or technique-level developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB offer deep Handle about operating processes and memory administration. Learning these equipment could possibly have a steeper learning curve but pays off when debugging efficiency difficulties, memory leaks, or segmentation faults.
Further than your IDE or debugger, turn out to be relaxed with Model Command systems like Git to comprehend code heritage, obtain the precise moment bugs were introduced, and isolate problematic adjustments.
Ultimately, mastering your resources signifies likely further than default settings and shortcuts — it’s about creating an intimate understanding of your advancement natural environment to make sure that when challenges crop up, you’re not shed in the dark. The greater you know your tools, the greater time you could expend resolving the particular challenge in lieu of fumbling by the method.
Reproduce the challenge
The most vital — and often ignored — actions in efficient debugging is reproducing the problem. Before leaping to the code or producing guesses, developers have to have to make a regular atmosphere or scenario where by the bug reliably seems. Without having reproducibility, fixing a bug results in being a video game of possibility, frequently leading to squandered time and fragile code modifications.
The initial step in reproducing a challenge is gathering just as much context as you can. Inquire thoughts like: What actions led to The difficulty? Which surroundings was it in — development, staging, or output? Are there any logs, screenshots, or error messages? The greater detail you have got, the less complicated it gets to be to isolate the precise situations under which the bug takes place.
After you’ve gathered adequate information, try and recreate the issue in your neighborhood atmosphere. This might mean inputting precisely the same information, simulating related person interactions, or mimicking program states. If The difficulty appears intermittently, take into account creating automatic checks that replicate the edge situations or point out transitions concerned. These assessments don't just assist expose the situation but also avert regressions Down the road.
Occasionally, The problem can be atmosphere-distinct — it'd happen only on specific running units, browsers, or under certain configurations. Working with tools like virtual machines, containerization (e.g., Docker), or cross-browser screening platforms might be instrumental in replicating these types of bugs.
Reproducing the trouble isn’t merely a action — it’s a mentality. It requires patience, observation, as well as a methodical technique. But when you finally can continuously recreate the bug, you might be already halfway to fixing it. With a reproducible scenario, You should use your debugging equipment a lot more properly, examination likely fixes safely and securely, and converse additional Evidently with your staff or users. It turns an abstract complaint into a concrete challenge — Which’s in which developers thrive.
Read through and Recognize the Mistake Messages
Error messages are frequently the most useful clues a developer has when anything goes Erroneous. Rather then looking at them as frustrating interruptions, builders should learn to treat error messages as direct communications in the method. They often show you what precisely took place, in which it happened, and in some cases even why it took place — if you understand how to interpret them.
Begin by reading the information meticulously and in full. Lots of builders, especially when less than time strain, glance at the main line and right away begin earning assumptions. But deeper in the mistake stack or logs may lie the genuine root result in. Don’t just duplicate and paste error messages into search engines like google — browse and realize them first.
Split the mistake down into elements. Can it be a syntax error, a runtime exception, or maybe a logic error? Will it point to a certain file and line number? What module or operate brought on it? These issues can guide your investigation and position you toward the dependable code.
It’s also helpful to be familiar with the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java generally adhere to predictable designs, and learning to recognize these can significantly hasten your debugging course of action.
Some mistakes are obscure or generic, As well as in These situations, it’s crucial to examine the context where the mistake occurred. Examine linked log entries, enter values, and recent improvements within the codebase.
Don’t neglect compiler or linter warnings possibly. These generally precede bigger challenges and provide hints about opportunity bugs.
In the end, mistake messages usually are not your enemies—they’re your guides. Mastering to interpret them appropriately turns chaos into clarity, encouraging you pinpoint concerns speedier, cut down debugging time, and become a a lot more economical and confident developer.
Use Logging Properly
Logging is One of the more powerful tools within a developer’s debugging toolkit. When employed properly, it offers serious-time insights into how an software behaves, assisting you recognize what’s going on under the hood without needing to pause execution or stage with the code line by line.
A superb logging tactic starts off with figuring out what to log and at what level. Typical logging ranges contain DEBUG, Information, WARN, Mistake, and Lethal. Use DEBUG for thorough diagnostic data for the duration of advancement, Information for common activities (like profitable start off-ups), WARN for possible issues that don’t crack the application, Mistake for genuine troubles, and FATAL when the procedure can’t continue on.
Keep away from flooding your logs with excessive or irrelevant data. Far too much logging can obscure significant messages and slow down your system. Deal with critical occasions, point out improvements, input/output values, and critical final decision factors inside your code.
Format your log messages Plainly and constantly. Consist of context, which include timestamps, request IDs, and function names, so it’s simpler to trace issues in dispersed methods or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to track how variables evolve, what ailments are met, and what branches of logic are executed—all devoid of halting the program. They’re In particular beneficial in output environments in which stepping through code isn’t attainable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about harmony and clarity. Which has a effectively-believed-out logging tactic, you are able to decrease the time it's going to take to spot troubles, attain deeper visibility into your programs, and Enhance the In general maintainability and reliability of one's code.
Consider Similar to a Detective
Debugging is not just a specialized undertaking—it is a form of investigation. To efficiently establish and fix bugs, developers have to tactic the procedure similar to a detective solving a thriller. This frame of mind can help stop working complex concerns into workable pieces and adhere to clues logically to uncover the root result in.
Start by gathering evidence. Consider the indications of the issue: error messages, incorrect output, or efficiency troubles. The same as a detective surveys a criminal offense scene, accumulate just as much suitable information and facts as you can without jumping to conclusions. Use logs, exam cases, and user reports to piece together a clear photo of what’s occurring.
Following, kind hypotheses. Request oneself: What could possibly be leading to this conduct? Have any modifications recently been built into the codebase? Has this difficulty transpired just before beneath comparable situations? The goal should be to slim down options and establish likely culprits.
Then, examination your theories systematically. Make an effort to recreate the issue in a managed atmosphere. If you suspect a certain operate or component, isolate it and validate if The problem persists. Similar to a detective conducting interviews, question your code concerns and Enable the final results lead you nearer to the truth.
Pay back near attention to smaller specifics. Bugs often disguise inside the the very least anticipated places—just like a lacking semicolon, an off-by-one particular error, or maybe a race situation. Be extensive and affected person, resisting the urge to patch The difficulty with out thoroughly knowing it. Non permanent fixes could hide the true trouble, only for it to resurface later on.
Last of all, preserve notes on Anything you attempted and figured out. Equally as detectives log their investigations, documenting your debugging method can help you save time for long term difficulties and help Other individuals fully grasp your reasoning.
By considering just like a detective, builders can sharpen their analytical skills, strategy challenges methodically, and become more effective at uncovering hidden difficulties in complex methods.
Publish Assessments
Crafting tests is one of the best strategies to transform your debugging skills and General advancement effectiveness. Assessments not simply assistance catch bugs early but also serve as a safety Web that offers you confidence when creating adjustments to the codebase. A properly-analyzed software is simpler to debug as it helps you to pinpoint exactly where and when a problem occurs.
Start with unit tests, which focus on person functions or modules. These small, isolated tests can quickly expose whether a selected bit of logic is Doing the job as envisioned. Every time a take a look at fails, you quickly know in which to search, considerably reducing some time used debugging. Device exams are Particularly useful for catching regression bugs—challenges that reappear immediately after Earlier getting fixed.
Future, combine integration exams and stop-to-stop tests into your workflow. These assistance make sure several areas of your application work together effortlessly. They’re notably beneficial for catching bugs that happen in elaborate programs with numerous factors or expert services interacting. If one thing breaks, your tests can inform you which A part of the pipeline unsuccessful and beneath what circumstances.
Crafting exams also forces you to definitely Consider critically about your code. To test a feature appropriately, you'll need to be familiar with its inputs, anticipated outputs, and edge conditions. This amount of understanding Obviously leads to higher code composition and fewer bugs.
When debugging a concern, writing a failing examination that reproduces the bug is usually a powerful initial step. As soon as the check fails continually, you are able to target fixing the bug and observe your take a look at go when the issue is solved. This solution ensures that precisely the same bug doesn’t return Down the road.
In brief, composing assessments turns debugging from the frustrating guessing recreation right into a structured and predictable course of action—helping you catch a lot more bugs, more rapidly plus more reliably.
Take Breaks
When debugging a tricky concern, it’s effortless to be immersed in the situation—gazing your display screen for hrs, seeking Alternative following Remedy. But The most underrated debugging equipment is actually stepping absent. Getting breaks will help you reset your head, lower irritation, and infrequently see The difficulty from the new point of view.
When you are way too near to the code for way too prolonged, cognitive tiredness sets in. You could possibly start off overlooking clear problems or misreading code you wrote just several hours previously. In this particular condition, your brain becomes less economical at trouble-resolving. A short walk, a coffee crack, or maybe switching to a unique process for 10–quarter-hour can refresh your concentration. Quite a few developers report discovering the foundation of a challenge once they've taken time for you to disconnect, permitting their subconscious get more info the job done from the track record.
Breaks also assist prevent burnout, In particular for the duration of for a longer time debugging sessions. Sitting down in front of a monitor, mentally caught, is not only unproductive but will also draining. Stepping away allows you to return with renewed Electrical power plus a clearer state of mind. You might quickly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you ahead of.
In the event you’re trapped, a superb rule of thumb should be to set a timer—debug actively for forty five–60 minutes, then take a five–10 moment split. Use that time to maneuver about, extend, or do some thing unrelated to code. It may well sense counterintuitive, Specifically less than restricted deadlines, however it essentially leads to speedier and more effective debugging In the long term.
In brief, getting breaks isn't an indication of weak spot—it’s a smart approach. It gives your brain Place to breathe, improves your viewpoint, and helps you avoid the tunnel eyesight That always blocks your progress. Debugging can be a mental puzzle, and rest is a component of resolving it.
Learn From Every single Bug
Each individual bug you experience is much more than simply a temporary setback—It really is a possibility to grow like a developer. No matter if it’s a syntax mistake, a logic flaw, or even a deep architectural challenge, every one can teach you one thing precious if you make time to replicate and review what went Incorrect.
Commence by asking by yourself a handful of key concerns after the bug is fixed: What prompted it? Why did it go unnoticed? Could it have been caught earlier with improved practices like device testing, code reviews, or logging? The answers often reveal blind places in the workflow or understanding and help you build stronger coding habits going ahead.
Documenting bugs can even be an outstanding practice. Hold a developer journal or keep a log where you Be aware down bugs you’ve encountered, how you solved them, and Anything you acquired. Eventually, you’ll begin to see designs—recurring troubles or frequent errors—that you could proactively avoid.
In team environments, sharing Anything you've figured out from a bug together with your peers is often Specially effective. Regardless of whether it’s through a Slack information, a short write-up, or A fast information-sharing session, helping Many others stay away from the exact same difficulty boosts crew efficiency and cultivates a more robust Understanding society.
Far more importantly, viewing bugs as lessons shifts your mentality from stress to curiosity. Rather than dreading bugs, you’ll get started appreciating them as crucial parts of your growth journey. In the end, a lot of the ideal developers will not be those who compose perfect code, but people that constantly master from their blunders.
Eventually, Every bug you deal with adds a whole new layer to your ability established. So next time you squash a bug, take a instant to reflect—you’ll appear absent a smarter, far more able developer due to it.
Conclusion
Improving upon your debugging expertise usually takes time, apply, and persistence — though the payoff is huge. It helps make you a far more efficient, assured, and able developer. Another time you might be knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to be much better at Whatever you do.